이번엔 자식 필터를 이용해서 실렉터를 지정해보자.
▶ 자식 필터(Child filters) 종류 ◀
선택자 | 설명 |
:first-child | 부모의 첫 번째 자식인 모든 요소를 선택 |
:last-child | 부모의 마지막 번째 자식인 모든 요소를 선택 |
:first-of-type | 동일한 요소 이름의 형제 중 첫 번째 요소를 모두 선택 |
:last-of-type | 동일한 요소 이름의 형제 중 마지막 번째 요소를 모두 선택 |
:nth-child(n) | 부모의 n 번째 자식인 모든 요소를 선택 |
:nth-child(even) | 부모의 짝수 번째 자식인 모든 요소를 선택 |
:nth-child(odd) | 부모의 홀수 번째 자식인 모든 요소를 선택 |
:nth-child(Xn+Y) | 부모의 Y 번째 요소부터 시작선택하여 Xn 마다 요소를 전부 선택 |
:nth-last-child(n) | 부모의 마지막 요소로부터 n 번째 자식인 모든 요소를 선택 |
:nth-last-child(even) | 부모의 마지막 요소로부터 짝수 번째 자식인 모든 요소 선택 |
:nth-last-child(odd) | 부모의 마지막 요소로부터 홀수 번째 자식인 모든 요소 선택 |
:nth-last-child(Xn+Y) | 부모의 마지막 요소로부터 Y 번째 요소부터 시작선택하여 Xn 마다 요소를 전부 선택 |
:nth-of-type(n) | 동일 요소 이름의 형제중 n 번째 요소를 모두 선택 |
:nth-of-type(even) | 동일 요소 이름의 형제중 짝수 번째 요소를 모두 선택 |
:nth-of-type(odd) | 동일 요소 이름의 형제중 홀수 번째 요소를 모두 선택 |
:nth-of-type(Xn+Y) | 동일 요소 이름의 형제중 Y 번째 요소부터 시작선택하여 Xn 마다 요소를 전부 선택 |
:nth-last-of-type(n) | 동일 요소 이름의 형제중 마지막 번째로부터 n 번째 요소를 모두 선택 |
:nth-last-of-type(even) | 동일 요소 이름의 형제중 마지막 번째로부터 짝수 번째 요소를 모두 선택 |
:nth-last-of-type(odd) | 동일 요소 이름의 형제중 마지막 번째로부터 홀수 번째 요소를 모두 선택 |
:nth-last-of-type(Xn+Y) | 동일 요소 이름의 형제중 마지막 번째로부터 Y 번째 요소부터 시작선택하여 Xn 마다 요소를 전부 선택 |
자식 필터를 이용한 선택자의 종류가 엄청 많다.
자, 이제부터 하나씩 어떻게 동작되는지 파헤쳐보자!!
예제 HTML CODE
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<script src="https://code.jquery.com/jquery-3.1.0.js"></script>
<title>JS Bin</title>
<script>
$(document).ready(function(){
// 코드 넣는 곳
});
</script>
</head>
<body>
<div>
<p>1번 콘텐츠 </p>
<p>2번 콘텐츠 </p>
<p>3번 콘텐츠 </p>
<p>4번 콘텐츠 </p>
<p>5번 콘텐츠 </p>
</div>
<div>
<p>6번 콘텐츠</p>
<p>7번 콘텐츠</p>
<p>8번 콘텐츠</p>
<p>9번 콘텐츠</p>
</div>
<div>
<ul>
<li>10번 콘텐츠</li>
<li>11번 콘텐츠</li>
<li>12번 콘텐츠</li>
</ul>
<ul>
<li>13번 콘텐츠</li>
<li>14번 콘텐츠</li>
<li>15번 콘텐츠</li>
</ul>
</div>
<div>
<p>16번 콘텐츠</p>
<p>17번 콘텐츠</p>
<p>18번 콘텐츠</p>
<p>19번 콘텐츠</p>
<p>20번 콘텐츠</p>
<p>21번 콘텐츠</p>
<p>22번 콘텐츠</p>
</div>
<div>
<p>23번 콘텐츠</p>
<p>24번 콘텐츠</p>
<p>25번 콘텐츠</p>
<p>26번 콘텐츠</p>
</div>
</body>
</html>
▶ :first-child와 :last-child 사용법
$('div:first-child').css({'color':'red'}); // - 1번
$('div p:first-child').css({'color':'red'}); // - 2번
이 두 개 코드를 테스트를 해보고 어떻게 동작하는지 확인해보자.
둘 중 하나는 주석 처리하고 위 HTML 코드 중 // 코드 넣는 곳 주석처리되어 있는 곳에 위 2개 의 코드를 넣고
테스트를 하면 된다.
1번 결과 - $('div:first-child'). css({'color':'red'});
선택자가 div 요소 중 first-child 니까 첫 번째 div의 모든 자식이 다 선택되었다.
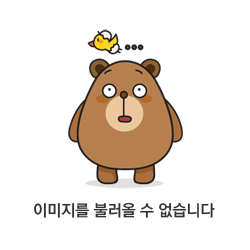
2번 결과 - $('div p:first-child'). css({'color':'red'});
div p 가 선택 자니까 각각 div 요소 중 p요소의 첫 번째 요소가 모두 선택되었다.
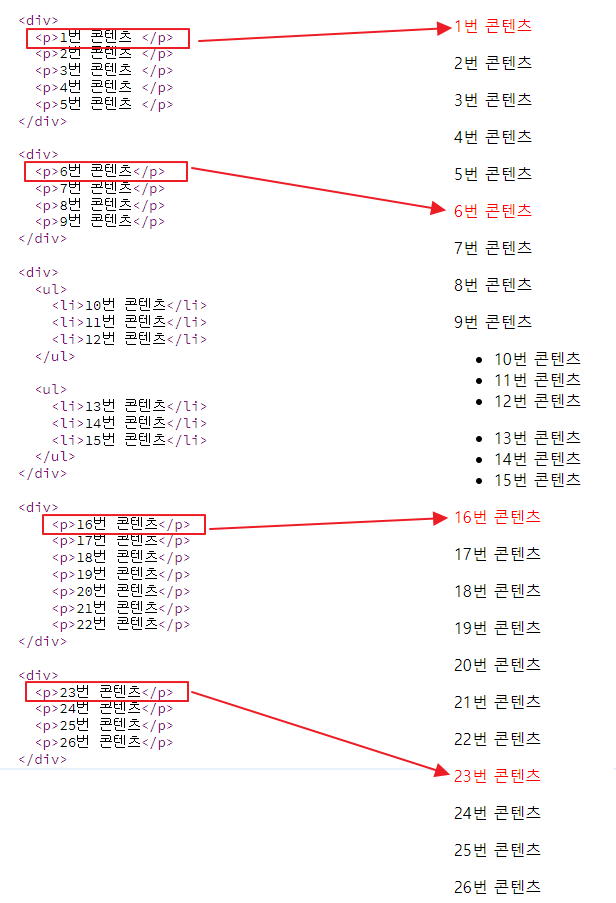
$('div:last-child').css({'color':'red'}); // - A
$('div p:last-child').css({'color':'red'}); // - B
A 결과 $('div:last-child'). css({'color':'red'});
당연히 마지막 div 요소의 모든 요소가 선택되었다.
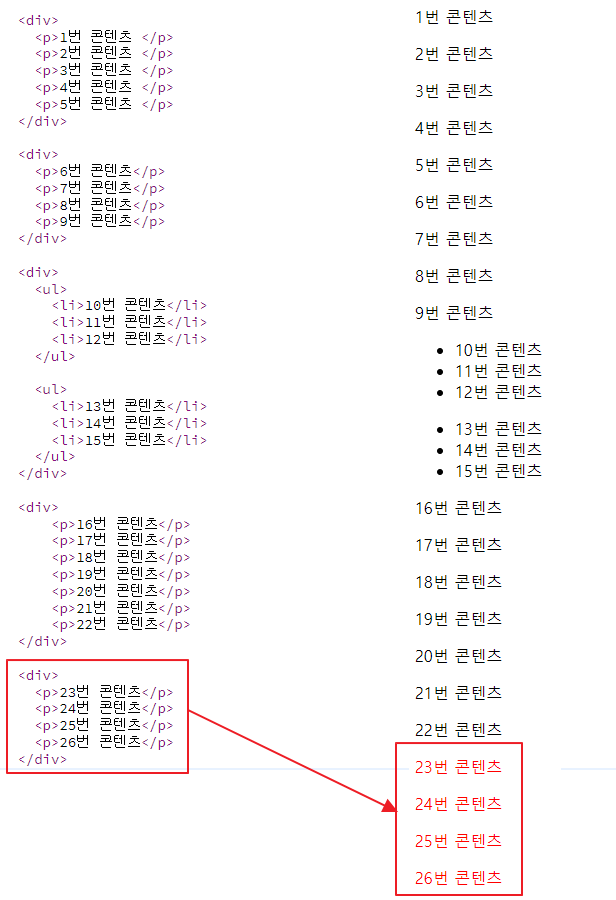
B 결과 $('div p:last-child'). css({'color':'red'});
각 div 요소 안에 p 요소의 마지막 번째의 요소들이 전부 선택되었다.
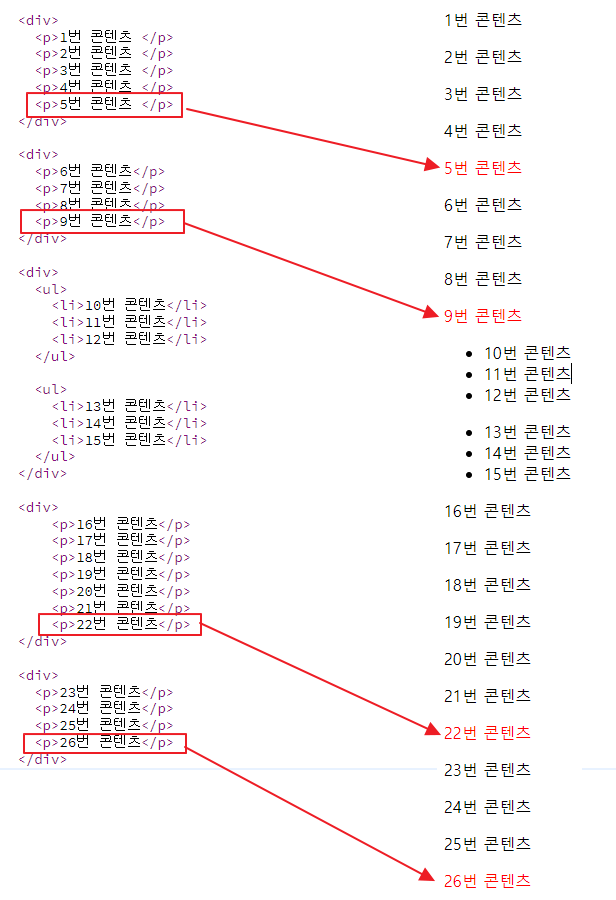
▶ :first-of-type와 :last-of-type 사용법
$('ul li:first-of-type').css({'color':'red'}); // - 1번
$('ul li:last-of-type').css({'color':'red'}); // - 2번
1번 결과 $('ul li:first-of-type'). css({'color':'red'});
동일 li 요소 중 첫 번째 요소 전부 선택되었다.
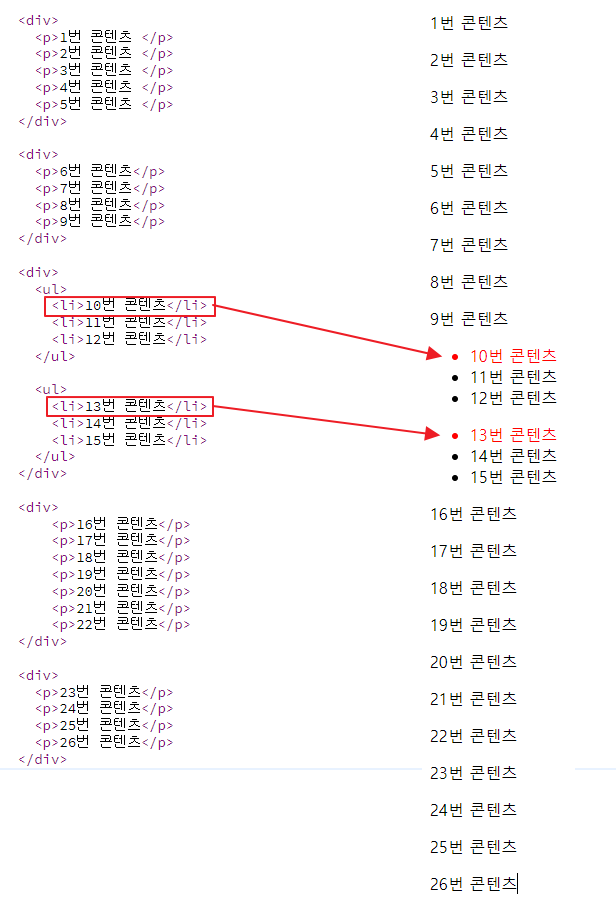
2번 결과 $('ul li:last-of-type'). css({'color':'red'});
동일 li 요소 중 마지막 번째 요소 전부 선택되었다.
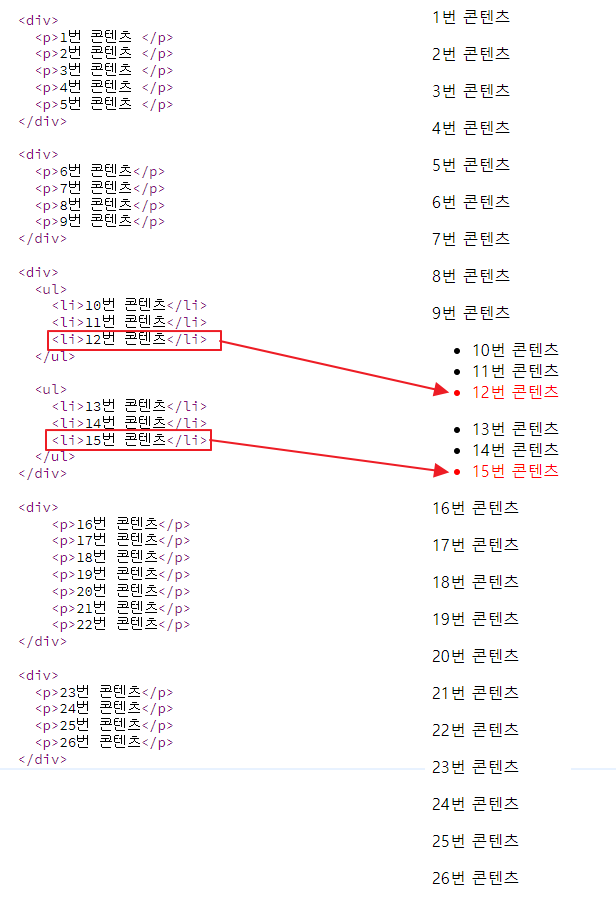
▶ :nth-child(n), :nth-child(even) , :nth-child(odd), :nth-child(Xn+Y) :nth-last-child(n), :nth-last-child(even), :nth-last-child(odd), :nth-last-child(Xn+Y) 사용법
의미는 같으나 처음 시작의 위치가 반대 | |
:nth-child(n) | :nth-last-child(n) |
:nth-child(even) | :nth-last-child(even) |
:nth-child(odd) | :nth-last-child(odd) |
:nth-child(Xn+Y) | :nth-last-child(Xn+Y) |
여기서는 빨간색 글자로 되어 있는 선택자들만 확인해보자.
행동하는 의미는 같으나 요소의 시작 위치가 반대라고 생각하면 된다.
:nth-child(n) 결과
$('div:nth-child(3)').css({'color':'red'}); // 실행 테스트 코드
선택자는 3번째 div 자식의 모든 요소를 선택되었다.
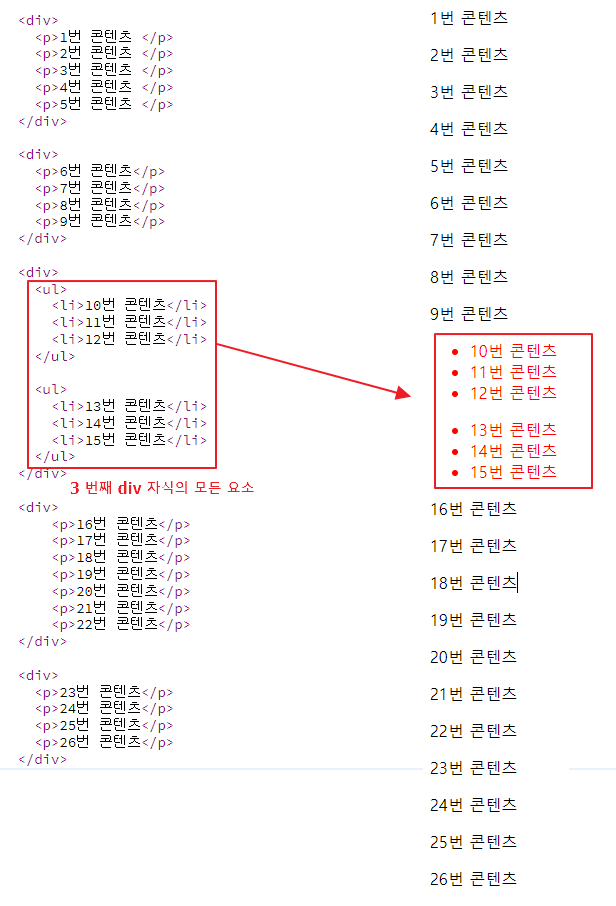
:nth-child(Xn+Y) 결과
$('div p:nth-child(3n+1)').css({'color':'red'}); // 실행 테스트 코드
테스트를 들어가기 전에 선택자가 어떻게 동작하는지 확인해보면, div의 p 태그 요소들 중
3n+1의 의미는 숫자 1은 첫 번째 요소를 포함 선택하여 그 후 3개의 요소마다 모두 선택 의미이다.
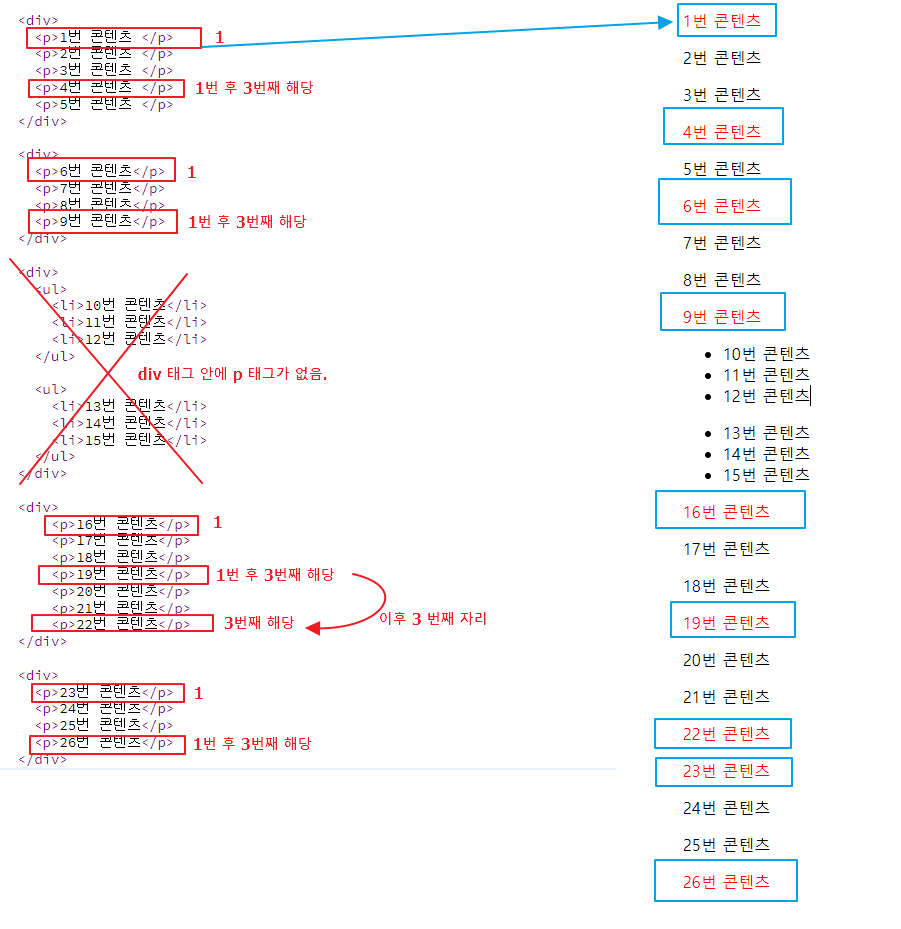
:nth-last-child(even) 결과
$('div:nth-last-child(even)').css({'color':'red'});
div 요소의 마지막 자리가 시작점 위치로부터 짝수의 div 자식 요소를 모두 선택되었다.
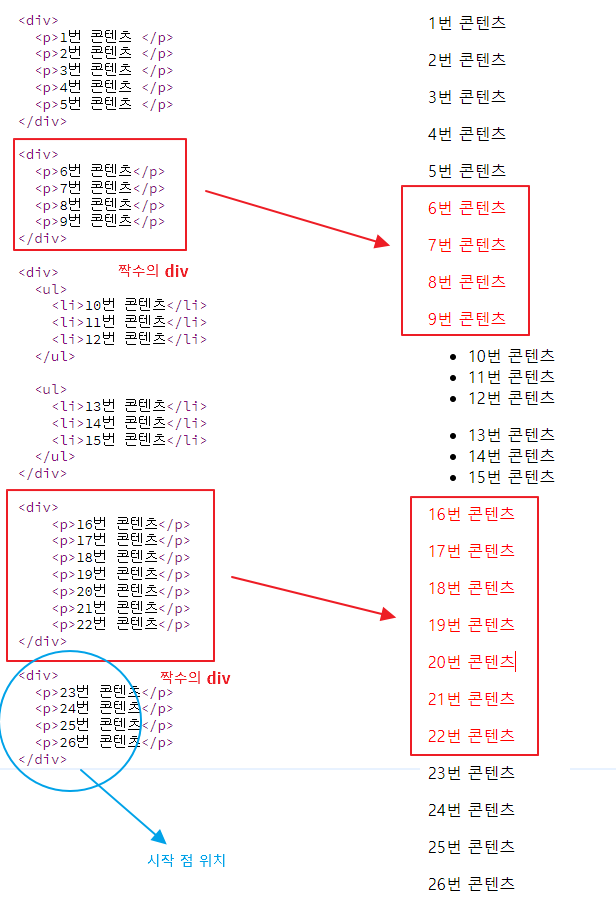
:nth-last-child(odd) 결과
$('div:nth-last-child(odd) ').css({'color':'red'});
div 요소의 마지막 자리가 시작점 위치로부터 홀수의 div 자식 요소를 모두 선택되었다.
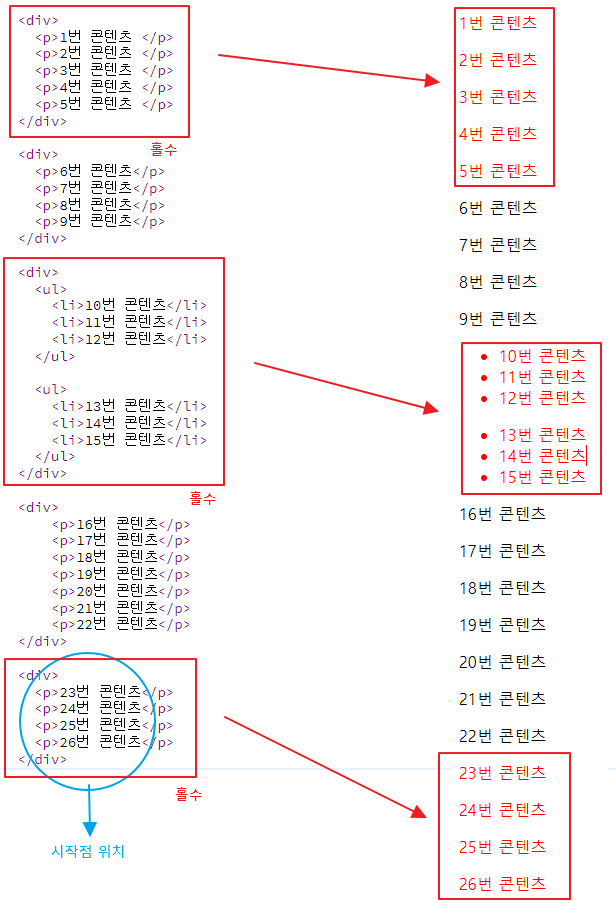
▶ :nth-of-type(n), :nth-of-type(even), :nth-of-type(odd), :nth-of-type(Xn+Y), :nth-last-of-type(n), :nth-last-of-type(even), :nth-last-of-type(odd), :nth-last-of-type(Xn+Y) 사용법
의미는 같으나 처음 시작의 위치가 반대 | |
:nth-of-type(n) | :nth-last-of-type(n) |
:nth-of-type(even) | :nth-last-of-type(even) |
:nth-of-type(odd) | :nth-last-of-type(odd) |
:nth-of-type(Xn+Y) | :nth-last-of-type(Xn+Y) |
이번 것도 빨간색 글자 선택자만 확인해보자. even, odd, Xn+Y 내용은 이 전과 동일하니
nth-of-type 이 선택자는 동일 요소의 형제라 생각하면 된다.
:nth-of-type(n) 결과
$('div:nth-of-type(2)').css({'color':'red'});
div 동일 요소중 2번째 모든 요소가 선택되었다.
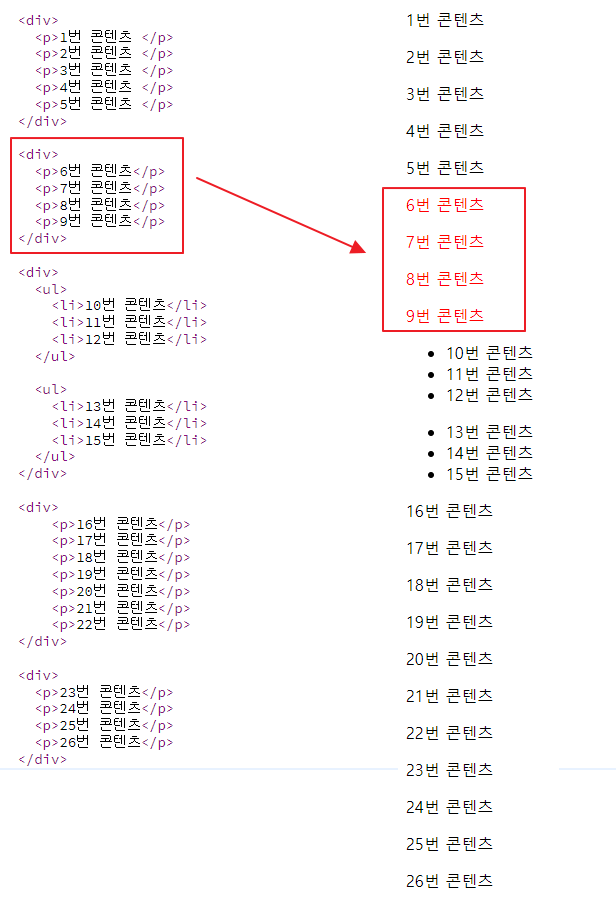
:nth-last-of-type(Xn+1) 결과
$('p:nth-last-of-type(2n+1)').css({'color':'red'});
p 태그 동일 모든 요소가 마지막 위치점 으로부터 (2n+1) 중에 1은 첫 번째 자리 선택 포함으로부터
시작하여 2마다 요소를 모두 선택되었다.
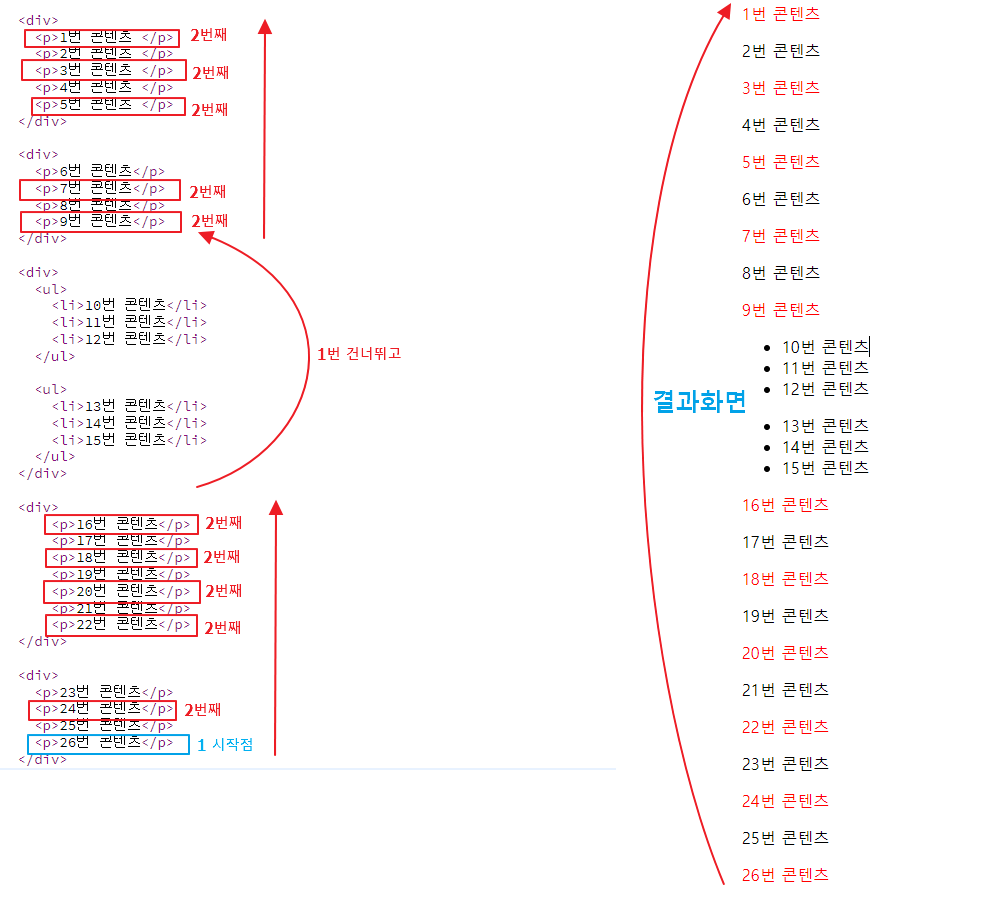
'web 언어 > jQuery' 카테고리의 다른 글
jQuery - 라디오(radio), 체크박스(checkbox) 클릭 된 요소 알기 (0) | 2022.03.15 |
---|---|
jQuery - DOM 요소 복제해서 다른 요소에 붙여넣기 (0) | 2022.03.15 |
jQuery - 셀렉터(selector) 선택자 (위치필터를 이용하여 요소 선택- first, last, even, odd, eq(), gt(), lt()) (0) | 2022.03.12 |
jQuery - 셀렉터(selector) 선택자 (속성을 이용하여 요소선택) (0) | 2022.03.12 |
jQuery - 셀렉터(selector) 선택자 (기본, 계층별 요소선택) (0) | 2022.03.11 |